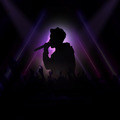
Gin是Go语言开发的web框架,它的性能很好,速度很快
下载安装就golang终端输入以下命令
go get -u github.com/gin-gonic/gin
下载速度可能较慢,我们可以在gomodule配置一下
使用的时候就像平时的模块一样导入 import 就可以
首先,我们可以创建大概的框架,基础的服务
package main
// gin 框架学习
import "github.com/gin-gonic/gin"
func main() {
// 创建服务
ginServer:=gin.Default()
// 1.访问地址,处理请求
// 可以发送get,post请求
ginServer.GET("/hello",func(context *gin.Context) {
context.JSON(200,gin.H {
"mes":"hello,world"})
})
// 2.服务器端口 如果不填的话是默认8080端口,这里填写则使用8082端口
ginServer.Run(":8082")
}
运行以上代码
[GIN-debug] [WARNING] Creating an Engine instance with the Logger and Recovery middleware already attached.
[GIN-debug] [WARNING] Running in "debug" mode. Switch to "release" mode in production.
- using env: export GIN_MODE=release
- using code: gin.SetMode(gin.ReleaseMode)
[GIN-debug] GET /hello --> main.main.func1 (3 handlers)
[GIN-debug] [WARNING] You trusted all proxies, this is NOT safe. We recommend you to set a value.
Please check https://pkg.go.dev/github.com/gin-gonic/gin#readme-don-t-trust-all-proxies for details.
[GIN-debug] Listening and serving HTTP on :8082
接下来,我们访问8082端口,在浏览器输入网址 localhost:8082/hello
因为,上面的请求是get请求,所以我们可以使用浏览器测试访问
这样就代表服务启动成功
我们也可以为这个网站添加一个图标
需要注意的是,网站图标必须是.iso文件
代码如下
package main
// gin 框架学习
import "github.com/gin-gonic/gin"
import "github.com/thinkerou/favicon"
func main() {
// 创建服务
ginServer:=gin.Default()
// 给打开的网址localhost:8082/hello 一个网址图标
ginServer.Use(favicon.New("./favicon.ico"))
// 1.访问地址,处理请求
// 可以发送get,post请求
ginServer.GET("/hello",func(context *gin.Context) {
context.JSON(200,gin.H {
"mes":"hello,world"})
})
// 2.服务器端口 如果不填的话是默认8080端口,这里填写则使用8082端口
ginServer.Run(":8082")
}
运行之后,
GOROOT=C:\Program Files\Go #gosetup
GOPATH=C:\Program Files\Go\bin;C:\Users\Admin\go #gosetup
"C:\Program Files\Go\bin\go.exe" build -o C:\Users\Admin\AppData\Local\Temp\GoLand\___go_build_gin_demo_demo1.exe gin_demo/demo1 #gosetup
C:\Users\Admin\AppData\Local\Temp\GoLand\___go_build_gin_demo_demo1.exe #gosetup
[GIN-debug] [WARNING] Creating an Engine instance with the Logger and Recovery middleware already attached.
[GIN-debug] [WARNING] Running in "debug" mode. Switch to "release" mode in production.
- using env: export GIN_MODE=release
- using code: gin.SetMode(gin.ReleaseMode)
[GIN-debug] GET /hello --> main.main.func1 (4 handlers)
[GIN-debug] [WARNING] You trusted all proxies, this is NOT safe. We recommend you to set a value.
Please check https://pkg.go.dev/github.com/gin-gonic/gin#readme-don-t-trust-all-proxies for details.
[GIN-debug] Listening and serving HTTP on :8082
[GIN] 2022/11/27 - 15:47:26 | 200 | 300.4µs | ::1 | GET "/hello"
[GIN] 2022/11/27 - 15:47:27 | 200 | 1.199ms | ::1 | GET "/favicon.ico"
再次输入localhost网址
get /user
post /create_user
post/update_user
post/delete_user
get / user
post / user
put /user
delete/user
package main
// gin 框架学习
import "github.com/gin-gonic/gin"
import "github.com/thinkerou/favicon"
func main() {
// 创建服务
ginServer:=gin.Default()
// 给打开的网址localhost:8082/hello 一个网址图标
ginServer.Use(favicon.New("./favicon.ico"))
// 1.访问地址,处理请求
// 可以发送get,post请求
ginServer.GET("/hello",func(context *gin.Context) {
context.JSON(200,gin.H {
"mes":"hello,world"})
})
// post 请求不能用浏览器测试,要使用postman等工具
ginServer.POST("/user",func(context *gin.Context) {
context.JSON(200,gin.H {
"mes":"post,user"})
})
// 2.服务器端口 如果不填的话是默认8080端口,这里填写则使用8082端口
ginServer.Run(":8082")
}
运行代码之后,在postman中
这样就代表测试成功
下面我们可以响应一个网页
package main
// gin 框架学习
import "github.com/gin-gonic/gin"
import "github.com/thinkerou/favicon"
func main() {
// 创建服务
ginServer:=gin.Default()
// 给打开的网址localhost:8082/hello 一个网址图标
ginServer.Use(favicon.New("./favicon.ico"))
// 加载静态页面 Glob 加载全局
ginServer.LoadHTMLGlob("templates/*")
// 1.访问地址,处理请求
// 可以发送get,post请求
//ginServer.GET("/hello",func(context *gin.Context) {
// context.JSON(200,gin.H {
// "mes":"hello,world"})
//})
// 响应一个页面给前端
ginServer.GET("index",func(c *gin.Context) {
c.HTML(200,"index.html",gin.H {"msg":"后台数据"})
})
// post 请求不能用浏览器测试,要使用postman等工具
//ginServer.POST("/user",func(context *gin.Context) {
// context.JSON(200,gin.H {
// "mes":"post,user"})
//})
////put delete
//ginServer.PUT("/user")
//ginServer.DELETE("/user")
// 2.服务器端口 如果不填的话是默认8080端口,这里填写则使用8082端口
ginServer.Run(":8082")
}
同时创建文件
<html lang="en">
<head>
<meta charset="UTF-8">
<title>我的第一个go web 网页</title>
</head>
<body>
<h1> 艾欧尼亚 昂扬不灭 </h1>
获取后端的数据为
{{.msg}}
</body>
</html>
运行上面代码
[GIN-debug] [WARNING] Creating an Engine instance with the Logger and Recovery middleware already attached.
[GIN-debug] [WARNING] Running in "debug" mode. Switch to "release" mode in production.
- using env: export GIN_MODE=release
- using code: gin.SetMode(gin.ReleaseMode)
[GIN-debug] Loaded HTML Templates (2):
-
- index.html
[GIN-debug] GET /index --> main.main.func1 (4 handlers)
[GIN-debug] [WARNING] You trusted all proxies, this is NOT safe. We recommend you to set a value.
Please check https://pkg.go.dev/github.com/gin-gonic/gin#readme-don-t-trust-all-proxies for details.
[GIN-debug] Listening and serving HTTP on :8082
[GIN] 2022/11/27 - 17:19:06 | 200 | 1.6342ms | ::1 | GET "/index"
Process finished with the exit code -1073741510 (0xC000013A: interrupted by Ctrl+C)
在浏览器中输入localhost:8082/index
响应成功!
文章评论